Machine Learning is a subfield of AI that uses statistical techniques to give computers the ability to sense patterns and make predictions.
It is a form of artificial intelligence that has been around for decades. But in recent years it has become more popular due to the improvements in computing power and data storage. The goal of machine learning is to automate tasks that are currently done by humans by teaching machines how to learn from data without being explicitly programmed how to do them. Its goal is to build systems that can automatically improve themselves with experience.
Many people think that doing machine learning in PHP is not possible but that’s not correct. Thanks to the php-ml library. php-ml is a machine learning library for PHP. With the help of php-ml you can train, create, import, export your machine learning models.
In this article, you will learn how to use php-ml to do machine learning in PHP. We will also see how to train our model and get predictions using PHP.
PHP-ML Library
To get started, you need to download composer in your system. Goto this link: COMPOSER DOWNLOAD
Now run this command inside the folder where you are going to create your PHP file.
composer require php-ai/php-ml
You can see php-ml documentation from here.
Data To Feed
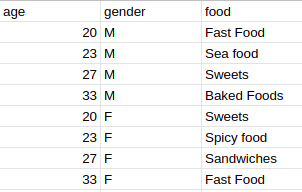
It is a CSV file. You can see there are three columns. 1-age, 2-gender & 3-food. According to the given data in csv 20 age male likes Fast Food, 23 age male likes Sea food, similarly 20 age female like Sweets, 23 age female like Spicy food and so on. We will use this data to train our model later in this article.
Our objective is to get the favorite food by giving age and gender to our machine learning model, which means age and gender will be our inputs and food will be our output. This is what we are going to learn.
Download this CSV file from here.
Machine Learning in PHP Code
Now create an index.php file and write this code. In the below code we have imported php-ml library. In our program, we need two classes ‘CsvDataset’ & ‘DecisionTree’. To access these classes we need to first use them in our code.
<?php
require_once __DIR__ . '/vendor/autoload.php';
use Phpml\Dataset\CsvDataset;
use Phpml\Classification\DecisionTree;
?>
The next step is to import the csv file. It’s super easy with php-ml.
/*
CsvDataset arguments :
1 - CSV File path
2 - Total columns in csv file
3 - Boolean value, if csv file has a heading row (if true then first row will be ignored)
*/
$dataset = new CsvDataset('foodml.csv', 3, true);
Now if you want to see CSV data, all you need is to call CsvDataset ‘getSamples()’ method. See the below code :
$samples = $dataset->getSamples();
//echo <pre> tag for clean output.
echo "<pre>";
print_r($samples);
The output will be this. All CSV rows are converted to a PHP array.
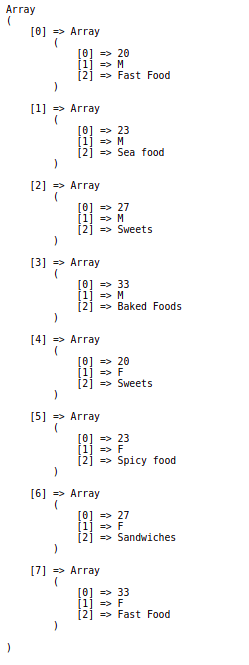
Now we need to separate the input & output dataset to train our model. age & gender are the input dataset and food is the output dataset. To separate them we need to create custom methods. See the below code where I created a class and added two methods.
class DataSetClass{
public function removeColumns(array $dataset, array $cols){
foreach ($dataset as &$row) {
foreach($row as $val){
foreach($cols as $index){
unset($row[$index]);
}
}
}
return $dataset;
}
public function getColumn(array $dataset ,int $colIndex){
$col = [];
foreach ($dataset as $e) {
$col[] = $e[$colIndex];
}
return $col;
}
}
The removeColumns() method takes two parameters, one is the dataset and the second one is the columns index number. Keep in mind in our csv there were three columns age, gender & food, where age index is 0, the gender index is 1, and the food index is 2. This method returns an array after removing all the rows of the given column index.
The getColumn() method also takes two parameters, one is the dataset and the second one is the column index number. This method will return an array with all the rows of a given column index number.
Now we need to use these two methods in our program to separate input and output datasets.
//Creating DataSetClass instance to use its methods
$dsClass = new DataSetClass();
//In input dataset, we need to remove food column because its an output dataset.
$input = $dsClass->removeColumns($samples, [2]);
//In output dataset, we need to get only food column data.
$output = $dsClass->getColumn($samples, 2);
READ: Creating a simple PHP routing with parameters.
Our input and output dataset is ready ! Now we need to train our model which is again an easy task.
//Create DecisionTree instance. This class is available in php-ml
$model = new DecisionTree();
//Train our model by feeding input and output.
$model->train($input, $output);
//Get predictions
//here we are giving 2 inputs. 25, M and 30, F.
var_dump($model->predict([[25, 'M'], [30, 'F']]));
//Output will be :
array(2) {
[0]=>
string(8) "Sea food"
[1]=>
string(10) "Sandwiches"
}
If you remember the CSV data, there were no 25 age male and 30 age female rows, but still, we got the predictions because this is what machine learning does. So according to our trained model 25 years old male like Sweets and 30 years old female like Sandwiches. The more data you feed to your model the better predictions you will get.
This is our final code :
<?php
require_once __DIR__ . '/vendor/autoload.php';
use Phpml\Dataset\CsvDataset;
use Phpml\Classification\DecisionTree;
class DataSetClass{
public function removeColumns(array $dataset, array $cols){
foreach ($dataset as &$row) {
foreach($row as $val){
foreach($cols as $index){
unset($row[$index]);
}
}
}
return $dataset;
}
public function getColumn(array $dataset ,int $colIndex){
$col = [];
foreach ($dataset as $e) {
$col[] = $e[$colIndex];
}
return $col;
}
}
echo "<pre>";
$dataset = new CsvDataset('foodml.csv', 3, true);
$samples = $dataset->getSamples();
$dsClass = new DataSetClass();
$input = $dsClass->removeColumns($samples, [2]);
$output = $dsClass->getColumn($samples, 2);
$model = new DecisionTree();
$model->train($input, $output);
var_dump($model->predict([[25, 'M'], [30, 'F']]));
?>
This is a very basic program of machine learning in PHP. To learn more follow the official docs of php-ml.