Expo is a free cross-platform development environment for prototyping and sharing your React Native apps on iOS and Android.It has a sleek design, is easy to use, and supports many native features. Expo provides many different tools for creating modern apps. Today we are going to see how we can rotate expo video player to full-screen mode.
I have seen many people trying to find a way to rotate expo video player in landscape mode. When you click on the full-screen button of expo video player, it becomes full screen but in portrait mode. Now the question is how we can rotate it to the landscape when someone clicks on the video player’s full-screen button?
Sounds difficult? but it’s very easy. I will tell you all the things step by step so don’t worry. Before starting make sure you have expo CLI installed on your system. Let create a new project with expo CLI.
//Start a new project
expo init video-player
cd video-player
expo start
STEP 1
We need two expo packages for our project. Install them with the below command
//Expo Video Player
expo install expo-av
//Expo Screen Orientation
expo install expo-screen-orientation
Docs on expo-av and expo-screen-orientation
STEP 2
Now create a simple App component with some basic styling and import required packages.
import { StatusBar } from 'expo-status-bar';
import React from 'react';
//Importing 'Dimensions' is important.
import { StyleSheet, View, Dimensions } from 'react-native';
//Import these packages.
import { Video } from 'expo-av';
import * as ScreenOrientation from 'expo-screen-orientation';
export default function App() {
return (
<View style={styles.container}>
<StatusBar style="auto" />
</View>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
backgroundColor: '#fff',
alignItems: 'center',
justifyContent: 'center',
},
});
STEP 3
Create a function that will be called when someone clicks on the video player’s fullscreen button.
function setOrientation() {
}
When the phone is in portrait/vertical mode, the height of the phone will be greater than the width of the phone and when the phone is in landscape/horizontal mode, the width of the phone will be greater than the height of the phone. See the below image you will understand it better.
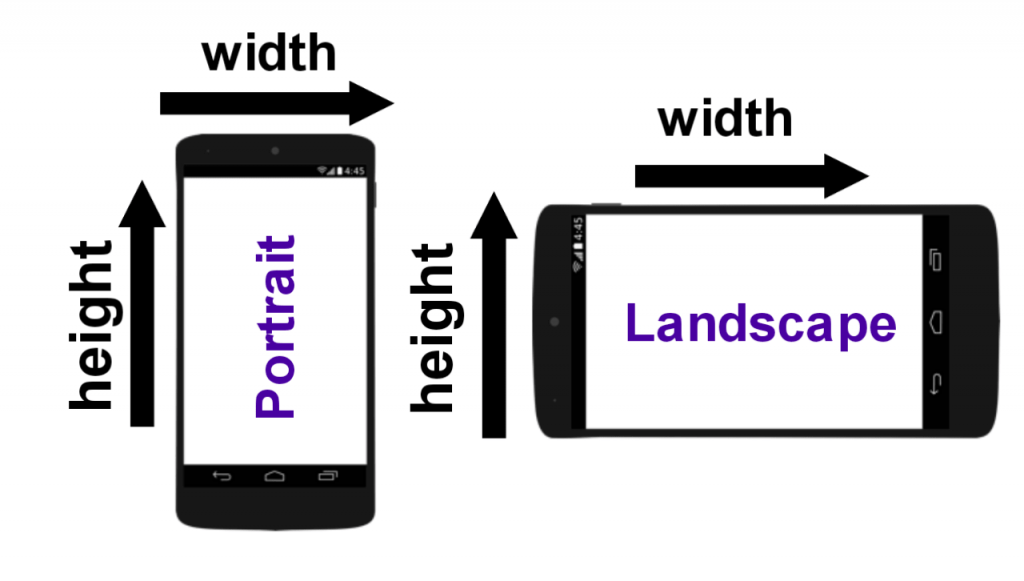
So by this condition, we can check whether the device is in portrait mode or in landscape mode. In react native Dimensions.get(‘window’).height will return the height of the device and Dimensions.get(‘window’).width will return the width of the device.
if (Dimensions.get('window').height > Dimensions.get('window').width) {
//Device is in portrait mode
}else{
//Device is in landscape mode
}
Remember we installed the “expo-screen-orientation” package? Now it’s time to use it to rotate the screen.
//Basic Usage of expo-screen-orientation
//Rotate screen to landscape mode
ScreenOrientation.lockAsync(ScreenOrientation.OrientationLock.LANDSCAPE);
//Rotate screen to portrait mode
ScreenOrientation.lockAsync(ScreenOrientation.OrientationLock.PORTRAIT);
So our function setOrientation() should look like this :
function setOrientation() {
if (Dimensions.get('window').height > Dimensions.get('window').width) {
//Device is in portrait mode, rotate to landscape mode.
ScreenOrientation.lockAsync(ScreenOrientation.OrientationLock.LANDSCAPE);
}
else {
//Device is in landscape mode, rotate to portrait mode.
ScreenOrientation.lockAsync(ScreenOrientation.OrientationLock.PORTRAIT);
}
}
STEP 4
Now add the Video component inside the main View. Don’t forget to pass onFullscreenUpdate prop with setOrientation() function. When full-screen button gets clicked, setOrientation() function will be called.
<Video
source={{ uri: 'http://d23dyxeqlo5psv.cloudfront.net/big_buck_bunny.mp4'}}
resizeMode="cover"
shouldPlay
onFullscreenUpdate={setOrientation}
useNativeControls
style={{ width: Dimensions.get('window').width, height: 200 }}
/>
So our final code will look like this :
import { StatusBar } from 'expo-status-bar';
import React from 'react';
import { StyleSheet, View, Dimensions } from 'react-native';
import { Video } from 'expo-av';
import * as ScreenOrientation from 'expo-screen-orientation';
export default function App() {
function setOrientation() {
if (Dimensions.get('window').height > Dimensions.get('window').width) {
//Device is in portrait mode, rotate to landscape mode.
ScreenOrientation.lockAsync(ScreenOrientation.OrientationLock.LANDSCAPE);
}
else {
//Device is in landscape mode, rotate to portrait mode.
ScreenOrientation.lockAsync(ScreenOrientation.OrientationLock.PORTRAIT);
}
}
return (
<View style={styles.container}>
<StatusBar style="auto" />
<Video
source={{ uri: 'http://d23dyxeqlo5psv.cloudfront.net/big_buck_bunny.mp4' }}
resizeMode="cover"
shouldPlay
onFullscreenUpdate={setOrientation}
useNativeControls
style={{ width: Dimensions.get('window').width, height: 200 }}
/>
</View>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
backgroundColor: '#fff',
alignItems: 'center',
justifyContent: 'center',
},
});
This is how you can rotate the expo video player in full-screen landscape mode. I hope you understood this. If you have any questions feel free to comment, I will be happy to answer.
I have also made a react-native component for this check here.