Reviews and ratings are one of the most important parts for the users to understand and trust a product, an app, a movie, or a seller available in your app. Review is one of the most common features for an eCommerce app and to be honest without reviews feature one’s eCommerce business cannot get success. Reviews help others to understand a product in a better way and they also help to gain user’s trust.
What if a product has hundreds of reviews? Would your users read all reviews? Definitely not! Here ratings come, ratings provide a simple 5 – 10 stars scale that users can easily count and predict how good or bad a product is.
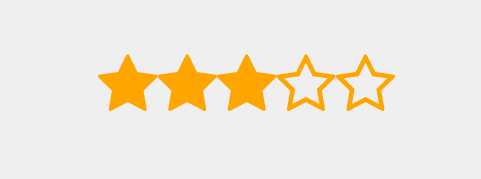
Today, we are going to create a simple star ratings in react native without using any third-party library. We will be using only Material Icons. If you have created react native project by using expo CLI then you don’t need to install any packages else if you are using create-react-native-app you need to install Vector Icons by running this command :
npm i react-native-vector-icons
You can know more about React Native Vector icons from here.
Create New Project With Expo :
//Install Expo CLI if you don't have
npm install --global expo-cli
//Start a new project
expo init star-ratings
cd star-ratings
expo start
Star ratings in React Native
Let’s start by creating a simple View and importing all the required components.
import * as React from 'react';
import { View, StyleSheet, Text } from 'react-native';
import { MaterialIcons } from '@expo/vector-icons';
export default function App() {
return(
<View style={styles.container}>
</View>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
flexDirection : "row",
justifyContent: 'center',
alignItems : "center",
padding: 8,
},
});
So, I have defined an App component and added a View with some basic styling. Also, you can see I have imported material icons from ‘@expo/vector-icons’; , it is required. If you are not using expo cli then you need to import it like this :
import MaterialIcons from 'react-native-vector-icons/MaterialIcons';
Now we need two variables. The first one is to define total stars and the second one is to define gained stars.
const totalStars = 5;
const gainedStars = 3;
In ES6, you can run a loop with Array.from() method.
The Array.from()
static method creates a new, shallow-copied Array
instance from an array-like or iterable object. (from mozilla docs)
Array.from({length: 20}, (x, i) => i);
More Info : StackOverflow
So with the help of Array.from() we can do something like this to show more than one star. The first parameter in Array.from() method is an object where we defined length and the second parameter is a function. I am also passing three props to the MaterialIcons component. “key” is a unique key for each Material Icon, “name” is the name of Material Icon, “size” is the size of Material Icon, “color” is the color of Material Icon.
{
Array.from({length: 5}, (x, i) => {
return(
<MaterialIcons key={i} name="star" size={30} color="#FFA000"/>
)
})
}
You have understood Array.from(). Now the next step is very easy and simple. You will find two-star icons on material icons. One is a star with filled color second is a star with only a border and without any filled color.
Material star icon with filled color name is “star”, material star icon with border and without a filled color name is “star-border”.


{
Array.from({length: gainStars}, (x, i) => {
return(
<MaterialIcons key={i} name="star" size={30} color="#FFA000"/>
)
})
}
{
Array.from({length: totalStars-gainStars}, (x, i) => {
return(
<MaterialIcons key={i} name="star-border" size={30} color="#FFA000" />
)
})
}
The first Array.from() will create filled color stars and the length will be the total number of gained stars. The second Array.from() will create a bordered stars (without fill color) and the length will be (total stars – gained stars). Just a reminder “length” refers to the number of iteration.
Let’s say if the total stars are 5 and the gained stars are 3 it will make something like this :

The final code will look like this :
import * as React from 'react';
import { View, StyleSheet, Text } from 'react-native';
import { MaterialIcons } from '@expo/vector-icons';
export default function App() {
const totalStars = 5;
const gainStars = 3;
return (
<View style={styles.container}>
{
Array.from({length: gainStars}, (x, i) => {
return(
<MaterialIcons key={i} name="star" size={30} color="#FFA000"/>
)
})
}
{
Array.from({length: totalStars-gainStars}, (x, i) => {
return(
<MaterialIcons key={i} name="star-border" size={30} color="#FFA000" />
)
})
}
</View>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
flexDirection : "row",
justifyContent: 'center',
alignItems : "center",
padding: 8,
},
});
Final Look Of Our App
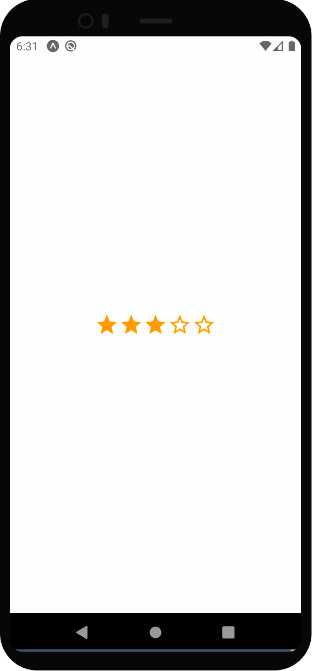
You can test this on your browser with expo snack. Go to this link -> Expo Snack Example